Easy way to deploy Linode instances across all (core) regions using Terraform
In preparations for a upcoming blog post related to running AI workloads on Akamai Connected Cloud, I needed to deploy instances in all available Linode regions. Since there’s no way that I’ll manually create a list of regions, I thought it would be cool to use Terraforms HTTP provider to dynamically fetch the list of regions and deploy an instance to it.
What’s the Plan?
- Fetch the list of regions: Use Terraform’s HTTP provider to retrieve the list of Linode regions via the Linode API.
- Deploy Instances: Use Terraform’s Linode provider to deploy instances in each of these regions.
- Grab some coffee: Sit back and relax while Terraform does the heavy lifting.
Pre-requisites
Before we begin, make sure you have:
- A Linode account (If not, sign up here).
- A Linode API token (Grab it from your Linode dashboard).
- Terraform installed on your machine (Download it from here).
Step-by-Step Guide
Step 1: Define Providers and Variables
We’ll start by defining our providers and variables. The HTTP provider will fetch the regions, and the Linode provider will deploy our instances.
provider "http" {}
variable "token" {
type = string
default = "mytoken" # Replace with your actual Linode API token
}
Step 2: Fetch Regions from Linode API.
Next, let’s fetch the list of regions using the HTTP provider. We’ll decode the JSON response to extract the region IDs.
Please note that if you uncomment the “request_headers” part, you will get a list of regions only available to your user/account. By default you will get a list of all public regions.
data "http" "regions" {
url = "https://api.linode.com/v4/regions"
# request_headers = {
# Authorization = "Bearer ${var.token}"
# }
}
locals {
regions = [for region in jsondecode(data.http.regions.response_body).data : region.id]
}
Step 3: Deploy Linode Instances
Now comes the fun part! We’ll utilize Terraform’s “for_each"
feature to loop through the regions and deploy a virtual machine.
provider "linode" {
token = var.token
}
resource "linode_instance" "instances" {
for_each = toset(local.regions)
label = "linode-${each.key}"
region = each.key
type = "g6-standard-1" # Example Linode type, adjust as needed
image = "linode/ubuntu20.04" # Example image, adjust as needed
root_pass = "your_secure_password" # Replace with a secure password
authorized_keys = [file("~/.ssh/id_rsa.pub")] # Adjust path to your public key
}
Step 4: Outputs
Finally, let’s define some outputs to see the regions and instances we’ve created.
output "regions" {
value = local.regions
}
output "instances" {
value = { for instance in linode_instance.instances : instance.id => instance }
}
Wrapping Up
And there you have it! With just a few lines of code, we’ve automated the deployment of Linode instances across all regions. Terraform takes care of the heavy lifting, allowing you to focus on what matters most – enjoying a cup of coffee while your infrastructure magically sets itself up :D+
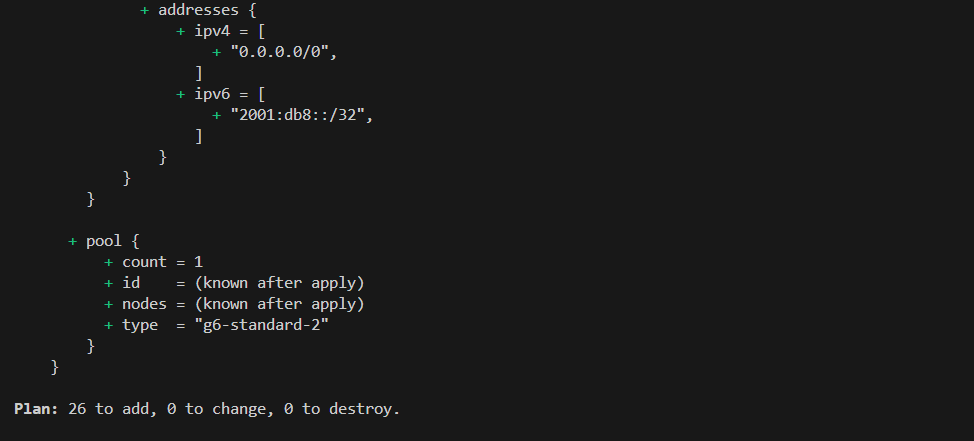
Until next time, Alex!